Garbage Mixer
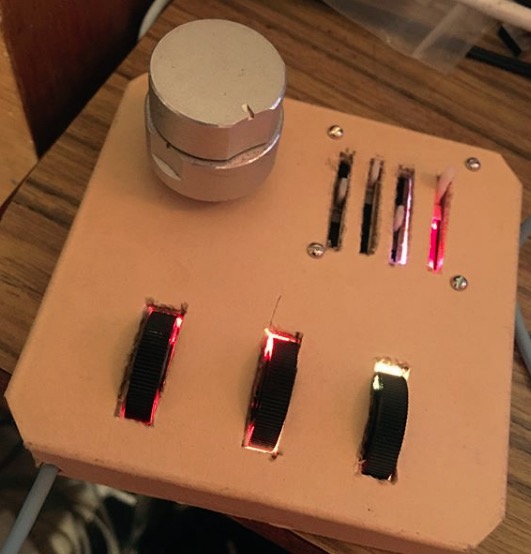
progress continues on my pet project garbage #interface#π all #salvage controls, vintage #univac wire, thicc #cardboard box.
when complete, video will be at @greasyconversation follow n live relatives β¨π¨π€ππ§
code for arduino pro micro / leonardo:
NOTE there’s a really fancy pong game easter egg in this one, with crazy modes where the paddles randomly come closer and the ball can become a bomb
// Woz Supposedly of Greasy Conversation - 11-21-18
#include <Keyboard.h>
#include <Mouse.h>
#include <MIDIUSB.h>
#include <Adafruit_NeoPixel.h>
#include <Arduino.h>
#include <U8g2lib.h>
#include <SPI.h>
#include <Wire.h>
U8G2_SSD1306_128X32_UNIVISION_F_HW_I2C u8g2(U8G2_R2);
#define outputA 16
#define outputB 15
#define pushpinF 7
#define mouse 3
#define scrl 1
char junk;
bool justwheel = false;
bool swap = true;
int mode = 2;
int maxBri = 255;
int aState;
int aLastState;
int goC;
int bState;
int bLastState;
int modecheck;
int mmodecheck;
int demo = 8;
int demod;
int space = 30;
int channel = 7; //starts with 0, so the real channel shows up as this number +1
int lastpatch = 0;
bool patchchange = false;
int r = 0;
int g = 0;
int b = 0;
int distchange = 33;
int dist = 0;
#define distfilter 15
int distchange2 = 33;
int dist2 = 0;
#define distfilter2 15
#define NUM_AI 6
#define ANALOGUE_PIN_ORDER A2, A3, A6, A8, A9, A10
#define MIDI_CC MIDI_CC_GENERAL1
Adafruit_NeoPixel strip = Adafruit_NeoPixel(8, 14, NEO_GRB + NEO_KHZ800);
#define xc 63
#define yc 16
int p1pad = 16;
int p2pad = 16;
int p1padchange = 16;
int p2padchange = 16;
int paddrift = 0;
int pspeed = 20;
int p1score = 0;
int p2score = 0;
int pballangle = 3;
int px = 16;
int py = 16;
#define pwin 9 ////----------rounds in game
#define popchance 10
#define driftchance 10
// #define bonuschance 200
// #define bonuspos 35
bool pbonus = 0;
int pvol = 0;
int pf = 1;
bool pop = false;
bool psensor = true;
bool pchill = false;
int line;
// A knob or slider movement must initially exceed this value to be recognised as an input. Note that it is
// for a 7-bit (0-127) MIDI value.
#define FILTER_AMOUNT 8
// Timeout is in microseconds
#define ANALOGUE_INPUT_CHANGE_TIMEOUT 600000
// Array containing a mapping of analogue pins to channel index. This array size must match NUM_AI above.
byte analogueInputMapping[6] = {ANALOGUE_PIN_ORDER};
byte analogueInputs[6];
byte tempAnalogueInput;
// Preallocate the for loop index so we don't keep reallocating it for every program iteration.
byte i = 0;
byte digitalOffset = 0;
// Variable to hold difference between current and new analogue input values.
byte analogueDiff = 0;
// This is used as a flag to indicate that an analogue input is changing.
boolean analogueInputChanging[6];
// Time the analogue input was last moved
unsigned long analogueInputTimer[6];
void controlChange(byte thechannel, byte control, byte value) {
midiEventPacket_t event = {0x0B, 0xB0 | thechannel, control, value};
MidiUSB.sendMIDI(event);
MidiUSB.flush();
}
// void pitchBendChange(byte thechannel, int value) {
// byte lowValue = value & 0x7F;
// byte highValue = value >> 7;
// midiEventPacket_t event = {0x0E, 0xE0 | thechannel, lowValue, highValue};
// MidiUSB.sendMIDI(event);
// MidiUSB.flush();
//}
void setup() {
Keyboard.begin();
Mouse.begin();
// Serial.begin(9600); // open the serial port at 9600 bps:
delay(2000);
u8g2.begin();
pinMode (outputA,INPUT_PULLUP);
pinMode (outputB,INPUT_PULLUP);
pinMode (pushpinF,INPUT_PULLUP);
pinMode(14, OUTPUT); //LED pin
pinMode(A0, INPUT);
pinMode(A1, INPUT);
pinMode(A2, INPUT);
pinMode(A3, INPUT);
pinMode(A6, INPUT);
// pinMode(A7, INPUT);
pinMode(A8, INPUT);
pinMode(A9, INPUT);
pinMode(A10, INPUT);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
strip.setBrightness(255);
}
void mousetext(){
u8g2.clearBuffer();
u8g2.setFont(u8g2_font_logisoso16_tr);
u8g2.setCursor(0, 16);
mmodecheck = analogRead(A9)/8;
if (mmodecheck<40){
mode=1;
u8g2.print("a r r o w");
}else if (mmodecheck>80){
mode=3;
u8g2.print("s c r o l l");
}else{
mode=2;
u8g2.print("m o u s e");
}
if(!swap){
u8g2.setFont(u8g2_font_6x12_tr);
u8g2.print(" ");
u8g2.print(char(94));
} else {
u8g2.print(" >");
}
u8g2.setFont(u8g2_font_6x12_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(80, 26);
u8g2.print("m o d e");
u8g2.sendBuffer(); // transfer internal memory to the display
}
void mouseMode(){
if (digitalRead(pushpinF)==LOW){
int mmodechange = analogRead(A9)/32;
delay(100);
while(digitalRead(pushpinF)==LOW){
modecheck=((analogRead(A2)/8)+(analogRead(A2)/8)+(analogRead(A2)/8))/3;
if(modecheck<40){
justwheel = true;
ledclear();
}else if(modecheck>115){
gameMode();
oled();
}else{
justwheel = false;
}
mousetext();
}
if ( (analogRead(A9)/32)==mmodechange ) {
if (swap){
swap = false;
}else{
swap = true;
}
mousetext();
}
delay(300);
}
aState = digitalRead(outputA); // Reads the "current" state of the outputA
// If the previous and the current state of the outputA are different, that means a Pulse has occured
if (aState != aLastState){
// If the outputB state is different to the outputA state, that means the encoder is rotating clockwise
if (digitalRead(outputB) != aState) {
goC++;
if (goC >= 2){
goC = 0;
if (mode == 1){
if (swap){
//left arrow
Keyboard.write(0xD8);
}else{
//down arrow
Keyboard.write(0xD9);
}
delay(2);
}
if (mode == 2){
if (swap){
Mouse.move(-mouse,0,0);
}else{
Mouse.move(0,mouse,0);
}
}
if (mode == 3){
if (swap){
Keyboard.press(0x81);
Mouse.move(0,0,-scrl);
Keyboard.release(0x81);
}else{
Mouse.move(0,0,-scrl);
}
}
}
} else {
goC--;
if (goC <= -1){
goC = 1;
if (mode == 1){
if (swap){
//right arrow
Keyboard.write(0xD7);
}else{
//up arrow
Keyboard.write(0xDA);
}
delay(2);
}
if (mode == 2){
if (swap){
Mouse.move(mouse,0,0);
}else{
Mouse.move(0,-mouse,0);
}
}
if (mode == 3){
if (swap){
Keyboard.press(0x81);
Mouse.move(0,0,scrl);
Keyboard.release(0x81);
}else{
Mouse.move(0,0,scrl);
}
}
}
}
delay(4);
}
aLastState = aState; // Updates the previous state of the outputA with the current state
if(justwheel){
mouseMode();
}
}
void loop() {
mouseMode();
for (i = 0; i < 6; i++)
{
// Read the analogue input pin, dividing it by 8 so the 10-bit ADC value (0-1023) is converted to a 7-bit MIDI value (0-127).
tempAnalogueInput = ( (analogRead(analogueInputMapping[i]) / 16) + (analogRead(analogueInputMapping[i]) / 16) + (analogRead(analogueInputMapping[i]) / 16) + (analogRead(analogueInputMapping[i]) / 16) )/2;
// Take the absolute value of the difference between the curent and new values
analogueDiff = abs(tempAnalogueInput - analogueInputs[i]);
// Only continue if the threshold was exceeded, or the input was already changing
if ((analogueDiff > 0 && analogueInputChanging[i] == true) || analogueDiff >= FILTER_AMOUNT)
{
// Only restart the timer if we're sure the input isn't 'between' a value
// ie. It's moved more than FILTER_AMOUNT
if (analogueInputChanging[i] == false || analogueDiff >= FILTER_AMOUNT)
{
// Reset the last time the input was moved
analogueInputTimer[i] = micros();
// The analogue input is moving
analogueInputChanging[i] = true;
}
else if (micros() - analogueInputTimer[i] > ANALOGUE_INPUT_CHANGE_TIMEOUT)
{
analogueInputChanging[i] = false;
}
// Only send data if we know the analogue input is moving
if (analogueInputChanging[i] == true)
{
// Record the new analogue value
analogueInputs[i] = tempAnalogueInput;
if (i>2){
strip.setPixelColor(6-(i-1), strip.Color(tempAnalogueInput*2,255-(tempAnalogueInput*2),0));
strip.show();
}
//normal sliders
if (tempAnalogueInput<5){tempAnalogueInput=0;}
controlChange(channel, space+i, tempAnalogueInput);
oled();
}
}
}
//end scan
//check sensor
int distcheck = (analogRead(A1)+analogRead(A1)+analogRead(A1))/3;
int distcheck2 = (analogRead(A0)+analogRead(A0)+analogRead(A0))/3;
if(distcheck<250){distcheck = 0;}
if(distcheck2<250){distcheck2 = 0;}
if((distcheck<distchange-distfilter)||(distcheck>distchange+distfilter)){
if(distcheck>1000){distcheck = 0;}
dist = ((distcheck-250)/4);
if(dist>127){dist=127;}
if(dist<0){dist=0;}
int dr = dist*5.8;
if(dr>255){dr=255;}
int dg = (dist*5.8)-255;
if(dg<0){dg=0;}
if(dg>255){dg=255;}
int db = (dist*5.8)-511;
if(db>255){db=255;}
if(db<0){db=0;}
strip.setPixelColor(0, strip.Color(dr,dg,db));
strip.setPixelColor(1, strip.Color(dr,dg,db));
strip.setPixelColor(2, strip.Color(dr,dg,db));
strip.show();
controlChange(channel, space+8, dist);
distchange = distcheck;
oleddist();
}else
//check sensor 2
if((distcheck2<distchange2-distfilter2)||(distcheck2>distchange2+distfilter2)){
if(distcheck2>1000){distcheck2 = 0;}
dist2 = ((distcheck2-250)/4);
if(dist2>127){dist2=127;}
if(dist2<0){dist2=0;}
int dr2 = dist2*5.8;
if(dr2>255){dr2=255;}
int dg2 = (dist2*5.8)-255;
if(dg2<0){dg2=0;}
if(dg2>255){dg2=255;}
int db2 = (dist2*5.8)-511;
if(db2>255){db2=255;}
if(db2<0){db2=0;}
strip.setPixelColor(3, strip.Color(dr2,dg2,db2));
strip.setPixelColor(4, strip.Color(dr2,dg2,db2));
strip.show();
controlChange(channel, space+9, dist2);
distchange2 = distcheck2;
oleddist();
}else{
splash();
}
//void loop
}
void splash(){
demod++;
if (demod>250){
demod=0;
demo++;
u8g2.clearBuffer(); // clear the internal memory
}
if (demo==0){
u8g2.drawPixel(random(96)+32,random(26));
}
if (demo==1){
int x = random(128);
int y = random(32);
int z = random(10);
u8g2.drawLine(x,y,x+z,y+z);
u8g2.drawLine(x+z,y,x,y+z);
}
if (demo==2){
u8g2.drawCircle(random(128),random(32),random(8), U8G2_DRAW_ALL);
}
if (demo==3){
u8g2.drawFrame(random(128),random(32),random(32),random(16));
}
if (demo==4){
int x = random(118)+5;
int y = random(22)+5;
int z = random(5);
u8g2.drawLine(x,y-z,x,y+z);
u8g2.drawLine(x-z,y,x+z,y);
}
if (demo==5){
int x = random(128);
int y = random(32);
u8g2.drawLine(x+random(12),y,x,y+random(12));
}
if (demo==6){
u8g2.drawPixel(random(128),random(32));
}
if (demo==7){
u8g2.drawLine(random(128),random(32),random(128),random(32));
}
if (demo==8){
u8g2.drawCircle(random(128),random(32),random(4), U8G2_DRAW_ALL);
}
if (demo==9){
u8g2.setFont(u8g2_font_logisoso16_tr); // BIG NUMBERS choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 14);
u8g2.print("woz");
u8g2.setCursor(0, 28);
u8g2.print("supposedly");
demo=0;
}
//splash end
u8g2.sendBuffer(); // transfer internal memory to the display
// delay(10);
}
void oleddist(){
if((dist>0)||(dist2>0)){
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_logisoso16_tr); // BIG NUMBERS choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(9, 32);
u8g2.drawCircle(xc, yc, 5, U8G2_DRAW_ALL);
u8g2.drawCircle(xc, yc, dist2/12, U8G2_DRAW_LOWER_LEFT);
u8g2.drawCircle(xc, yc, dist/12, U8G2_DRAW_LOWER_RIGHT);
u8g2.drawCircle(xc, yc, dist2/12+2, U8G2_DRAW_UPPER_LEFT);
u8g2.drawCircle(xc, yc, dist/12+2, U8G2_DRAW_UPPER_RIGHT);
u8g2.drawCircle(xc, yc, dist2/12+4, U8G2_DRAW_LOWER_LEFT);
u8g2.drawCircle(xc, yc, dist/12+4, U8G2_DRAW_LOWER_RIGHT);
u8g2.drawCircle(xc, yc, dist2/12+6, U8G2_DRAW_UPPER_LEFT);
u8g2.drawCircle(xc, yc, dist/12+6, U8G2_DRAW_UPPER_RIGHT);
u8g2.print(dist2);
u8g2.setCursor(90, 32);
u8g2.print(dist);
}
u8g2.sendBuffer(); // transfer internal memory to the display
}
void oled(){
if(tempAnalogueInput/8>0){
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(10, 16);
u8g2.print(tempAnalogueInput);
u8g2.setCursor(82, 16);
u8g2.print("ch");
u8g2.print(space+i);
u8g2.drawLine(4, 3, 4, 29 );
u8g2.drawLine(1, 31-(tempAnalogueInput/4), 7, 31-(tempAnalogueInput/4) );
u8g2.drawLine(1, 32-(tempAnalogueInput/4), 7, 32-(tempAnalogueInput/4) );
u8g2.drawLine(1, 33-(tempAnalogueInput/4), 7, 33-(tempAnalogueInput/4) );
}
u8g2.sendBuffer(); // transfer internal memory to the display
}
void gametext(){
u8g2.clearBuffer();
u8g2.setFont(u8g2_font_6x12_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 8);
if(pchill){
u8g2.print("c h i l l ");
}else{
u8g2.print("w o z ");
}
u8g2.print("p o n g");
if(psensor){
u8g2.print(" air");
}
u8g2.setFont(u8g2_font_logisoso16_tr);
u8g2.setCursor(0, 26);
}
void newround(){
pspeed = 20;
pvol = 0;
pf=1;
pop = false;
paddrift=0;
pbonus=0;
}
void gameMode(){
p1score = 0;
p2score = 0;
newround();
pballangle = 3;
px = 16;
py = 16;
ledclear();
checkpin1();
p1padchange = p1pad;
while((p1padchange > p1pad-2)&&(p1padchange < p1pad+2)){
gametext();
u8g2.print("player 1 start");
u8g2.sendBuffer();
checkpin1();
}
delay(200);
while(digitalRead(5)){ // while not pushing wheel---------------
int chilltest = analogRead(A9)/8;
if (chilltest>100){
pchill=false;
psensor=true;
} else if (chilltest>60){
pchill=true;
psensor=true;
} else if (chilltest>20){
pchill=true;
psensor=false;
} else {
pchill=false;
psensor=false;
}
delay(pspeed);
u8g2.clearBuffer(); // clear the internal memory
//move ball
if (pballangle==1){
px=px+pf;
py=py-2*pf;
}
if (pballangle==2){
px=px+2*pf;
py=py-2*pf;
}
if (pballangle==3){
px=px+2*pf;
py=py-pf;
}
if (pballangle==4){
px=px+2*pf;
py=py+pf;
}
if (pballangle==5){
px=px+2*pf;
py=py+2*pf;
}
if (pballangle==6){
px=px+pf;
py=py+2*pf;
}
if (pballangle==7){
px=px-pf;
py=py+2*pf;
}
if (pballangle==8){
px=px-2*pf;
py=py+2*pf;
}
if (pballangle==9){
px=px-2*pf;
py=py+pf;
}
if (pballangle==10){
px=px-2*pf;
py=py-pf;
}
if (pballangle==11){
px=px-2*pf;
py=py-2*pf;
}
if (pballangle==12){
px=px-pf;
py=py-2*pf;
}
if (pballangle==13){
px=px+2*pf;
}
if (pballangle==14){
px=px-2*pf;
}
//end moving
// bonuscheck();
//top bounce
if (py<1){
if (pballangle==10){
pballangle=9;
}
if (pballangle==11){
pballangle=8;
}
if (pballangle==12){
pballangle=7;
}
if (pballangle==1){
pballangle=6;
}
if (pballangle==2){
pballangle=5;
}
if (pballangle==3){
pballangle=4;
}
}
// bottom bounce
if (py>32){
if (pballangle==4){
pballangle=3;
}
if (pballangle==5){
pballangle=2;
}
if (pballangle==6){
pballangle=1;
}
if (pballangle==7){
pballangle=12;
}
if (pballangle==8){
pballangle=11;
}
if (pballangle==9){
pballangle=10;
}
}
if(paddrift>0){
paddrift=paddrift+1;
if (paddrift>30){
paddrift=30;
}
}
if (px<4+paddrift){
volup();
if(py==p1pad-5){
pballangle=1;
}
else if(py==p1pad-4){
pballangle=2;
}
else if(py==p1pad-3){
pballangle=2;
}
else if(py==p1pad-2){
pballangle=3;
}
else if(py==p1pad-1){
pballangle=3;
}
else if(py==p1pad){
pballangle=13;
}
else if(py==p1pad+1){
pballangle=4;
}
else if(py==p1pad+2){
pballangle=4;
}
else if(py==p1pad+3){
pballangle=5;
}
else if(py==p1pad+4){
pballangle=5;
}
else if(py==p1pad+5){
pballangle=6;
}
else if(((py>p1pad+5)||(py<p1pad-5))&&(!pop)){
p2score++;
if(p2score>pwin){
p2win();
}else{
for(line=1; line<10; line = line+2){
u8g2.drawCircle(4+paddrift, py, line, U8G2_DRAW_ALL);
delay(20);
u8g2.sendBuffer();
}
newround();
gametext();
p1miss();
}
}
if(pop){
u8g2.clearBuffer(); // clear the internal memory
if((py>p1pad+5)||(py<p1pad-5)){
pop=false;
popsurvive();
p2miss();
paddrift=0;
pbonus=0;
px=6;
}else{
popmessage();
for(line=1; line<100; line = line+4){
sparkle();
u8g2.drawCircle(4+paddrift, py, line, U8G2_DRAW_ALL);
u8g2.sendBuffer();
}
p1score--;
p2score++;
if(p2score>pwin){
p2win();
}else{
newround();
gametext();
p1miss();
}
}
}else{
if((random(1,popchance)==1)&&(!pchill)){
pop=true;
}
}
if((random(1,driftchance)==1)&&(!pchill)&&(!pbonus)){
paddrift=paddrift+1;
}
/*
if((random(1,bonuschance)==1)&&(!pchill)&&(paddrift<1)){
pbonus=1;
}
*/
}
if (px>123-paddrift){
volup();
if(py==p2pad-5){
pballangle=12;
}
else if(py==p2pad-4){
pballangle=11;
}
else if(py==p2pad-3){
pballangle=11;
}
else if(py==p2pad-2){
pballangle=10;
}
else if(py==p2pad-1){
pballangle=10;
}
else if(py==p2pad){
pballangle=14;
}
else if(py==p2pad+1){
pballangle=9;
}
else if(py==p2pad+2){
pballangle=9;
}
else if(py==p2pad+3){
pballangle=8;
}
else if(py==p2pad+4){
pballangle=8;
}
else if(py==p2pad+5){
pballangle=7;
}
if(((py>p2pad+5)||(py<p2pad-5))&&(!pop)){
p1score++;
if(p1score>pwin){
p1win();
}else{
for(line=1; line<20; line = line+4){
u8g2.drawCircle(124-paddrift, py, line, U8G2_DRAW_ALL);
u8g2.sendBuffer();
}
newround();
gametext();
p2miss();
}
}
if(pop){
u8g2.clearBuffer(); // clear the internal memory
if((py>p2pad+5)||(py<p2pad-5)){
pop=false;
popsurvive();
p1miss();
paddrift=0;
pbonus=0;
px=121;
}else{
popmessage();
for(line=1; line<200; line = line+8){
sparkle();
u8g2.drawCircle(12-paddrift, py, line, U8G2_DRAW_ALL);
u8g2.sendBuffer();
}
p2score--;
p1score++;
if(p1score>pwin){
p1win();
}else{
newround();
gametext();
p2miss();
}
}
}else{
if((random(1,popchance)==1)&&(!pchill)){
pop=true;
}
}
if((random(1,driftchance)==1)&&(!pchill)&&(!pbonus)){
paddrift=paddrift+1;
}
/*
if((random(1,bonuschance)==1)&&(!pchill)&&(paddrift<1)){
pbonus=1;
}
*/
}
// if ((pballangle<7)||(pballangle==13))
// {
checkpin2();
// }
// if ((pballangle>6)&&(pballangle!=13))
// {
checkpin1();
// }
u8g2.drawLine(0+paddrift, p1pad-2, 0+paddrift, p1pad+2 );
u8g2.drawLine(1+paddrift, p1pad-3, 1+paddrift, p1pad+3 );
u8g2.drawLine(2+paddrift, p1pad-2, 2+paddrift, p1pad+2 );
u8g2.drawLine(125-paddrift, p2pad-2, 125-paddrift, p2pad+2 );
u8g2.drawLine(126-paddrift, p2pad-3, 126-paddrift, p2pad+3 );
u8g2.drawLine(127-paddrift, p2pad-2, 127-paddrift, p2pad+2 );
/*
if(pbonus){
u8g2.drawLine(0+bonuspos, p2pad-2, 0+bonuspos, p2pad+2 );
u8g2.drawLine(1+bonuspos, p2pad-3, 1+bonuspos, p2pad+3 );
u8g2.drawLine(2+bonuspos, p2pad-2, 2+bonuspos, p2pad+2 );
u8g2.drawLine(125-bonuspos, p1pad-2, 125-bonuspos, p1pad+2 );
u8g2.drawLine(126-bonuspos, p1pad-3, 126-bonuspos, p1pad+3 );
u8g2.drawLine(127-bonuspos, p1pad-2, 127-bonuspos, p1pad+2 );
}
*/
if (pop){
u8g2.drawCircle(px, py, 3, U8G2_DRAW_ALL);
}else{
u8g2.drawDisc(px, py, 2, U8G2_DRAW_ALL);
}
u8g2.setFont(u8g2_font_6x12_tr);
if ((p1score<10)&&(p1score>-1)){
u8g2.setCursor(56, 10);
}else{
u8g2.setCursor(49, 10);
}
u8g2.print(p1score);
u8g2.setCursor(66, 10);
u8g2.print(p2score);
u8g2.setCursor(66, 30);
u8g2.print(pvol);
for(line=1; line<32; line = line+2){
u8g2.drawLine(63, line, 63, line);
}
u8g2.sendBuffer();
}
}
void p1miss(){
checkpin2();
p2padchange = p2pad;
u8g2.print("player 2 start");
u8g2.sendBuffer();
delay(1000);
while((p2padchange > p2pad-2)&&(p2padchange < p2pad+2)&&digitalRead(5)){
checkpin2();
}
if (p2pad>p2padchange){
pballangle=9;
}
if (p2pad<p2padchange){
pballangle=10;
}
py=p2pad;
px=121;
pop=false;
}
void p2miss(){
checkpin1();
p1padchange = p1pad;
u8g2.print("player 1 start");
u8g2.sendBuffer();
delay(1000);
while((p1padchange > p1pad-2)&&(p1padchange < p1pad+2)&&digitalRead(5)){
checkpin1();
}
if (p1pad>p1padchange){
pballangle=4;
}
if (p1pad<p1padchange){
pballangle=3;
}
py=p1pad;
px=6;
pop=false;
}
void p1win(){
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 16);
u8g2.print("player 1 WINS");
/*
for(line=1; line<200; line = line+4){
u8g2.drawCircle(127-paddrift, py, line, U8G2_DRAW_ALL);
winblast();
u8g2.sendBuffer();
}*/
ledclear();
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 16);
u8g2.print(p1score);
u8g2.setCursor(22, 16);
u8g2.print("< winner");
u8g2.setCursor(109, 16);
u8g2.print(p2score);
u8g2.setFont(u8g2_font_6x12_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 30);
u8g2.print("now player 2 starts");
u8g2.sendBuffer();
checkpin2();
p2padchange = p2pad;
while((p2padchange > p2pad-2)&&(p2padchange < p2pad+2)&&(digitalRead(5)==HIGH)){
sparkle();
checkpin2();
}
if (p2pad>p2padchange){
pballangle=9;
}
if (p2pad<p2padchange){
pballangle=10;
}
ledclear();
py=p2pad;
px=124;
p1score=0;
p2score=0;
newround();
}
void p2win(){
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 16);
u8g2.print("player 2 WINS");
/* for(line=1; line<200; line = line+4){
u8g2.drawCircle(124-paddrift, py, line, U8G2_DRAW_ALL);
winblast();
u8g2.sendBuffer();
}*/
ledclear();
u8g2.clearBuffer(); // clear the internal memory
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 16);
u8g2.print(p1score);
u8g2.setCursor(22, 16);
u8g2.print("winner >");
u8g2.setCursor(109, 16);
u8g2.print(p2score);
u8g2.setFont(u8g2_font_6x12_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 30);
u8g2.print("now player 1 starts");
u8g2.sendBuffer();
checkpin1();
p1padchange = p1pad;
while((p1padchange > p1pad-2)&&(p1padchange < p1pad+2)&&(digitalRead(5)==HIGH)){
sparkle();
checkpin1();
}
if (p1pad>p1padchange){
pballangle=4;
}
if (p1pad<p1padchange){
pballangle=3;
}
ledclear();
py=p1pad;
px=4;
p1score=0;
p2score=0;
newround();
}
void checkpin1(){
if (psensor){
int distcheck2 = analogRead(A0);
if(distcheck2<175){distcheck2 = 0;}
if((distcheck2<distchange2-distfilter2)||(distcheck2>distchange2+distfilter2)){
if(distcheck2>1000){distcheck2 = 0;}
dist2 = ((distcheck2-175)/4.25);
if(dist2>127){dist2=127;}
if(dist2<0){dist2=0;}
}
p1pad = (dist2/5)+3;
}else{
p1pad = ((33-(analogRead(A8)/34))+(33-(analogRead(A8)/34))+(33-(analogRead(A8)/34)))/3;
}
}
void checkpin2(){
if (psensor){
int distcheck = analogRead(A1);
if(distcheck<175){distcheck = 0;}
if((distcheck<distchange-distfilter)||(distcheck>distchange+distfilter)){
if(distcheck>1000){distcheck = 0;}
dist = ((distcheck-175)/4.25);
if(dist>127){dist=127;}
if(dist<0){dist=0;}
}
p2pad = (dist/5)+3;
}else{
p2pad = ((33-(analogRead(A10)/34))+(33-(analogRead(A10)/34))+(33-(analogRead(A10)/34)))/3;
}
}
void ledclear(){
for(int l=0; l<8; l++){
strip.setPixelColor(l, strip.Color(0,0,0));
}
strip.show();
}
void sparkle(){
int yeah = random(7);
strip.setPixelColor(yeah, strip.Color(255,255,255));
strip.show();
delay(10);
strip.setPixelColor(yeah, strip.Color(0,0,0));
strip.show();
}
void winblast(){
/*
int dr = line*7.5;
if(dr>255){dr=255;}
int dg = (line*7.5)-255;
if(dg<0){dg=0;}
if(dg>255){dg=255;}
int db = (line*7.5)-511;
if(db>255){db=255;}
if(db<0){db=0;}
for(int l=0; l<5; l++){
strip.setPixelColor(l, strip.Color(dr,dg,db));
}
strip.show();
delay(10);
*/
}
void popmessage(){
u8g2.setFont(u8g2_font_logisoso16_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 16);
u8g2.print("exploding ball!");
}
void popsurvive(){
u8g2.setFont(u8g2_font_6x12_tr); // choose a suitable font at https://github.com/olikraus/u8g2/wiki/fntlistall
u8g2.setCursor(0, 8);
u8g2.print("you survived!");
u8g2.setFont(u8g2_font_logisoso16_tr);
u8g2.setCursor(0, 26);
}
void volup(){
if((pvol>4)&&(!pchill)){pf=(pvol/4);}
pspeed--;
if (pspeed<0){pspeed=0;}
pvol++;
}
/*
void bonuscheck(){
if (pbonus){
if((px=129-bonuspos)&&((py<p1pad+5)&&(py>p1pad-5))) {
for(int n=1; n<6;n++){
if(pballangle==6+n){
pballangle=7-n;
}
}
if(pballangle==14){
pballangle=13;
}
px++;
}
if ((px=bonuspos-2) && ((py<p2pad+5)&&(py>p2pad-5))) {
for(int q=1; q<6;q++){
if(pballangle==q){
pballangle=13-q;
}
}
if(pballangle==13){
pballangle=14;
}
px--;
}
} //end bonus check
}
*/