Zoom GX1on Sync Pulse Mod
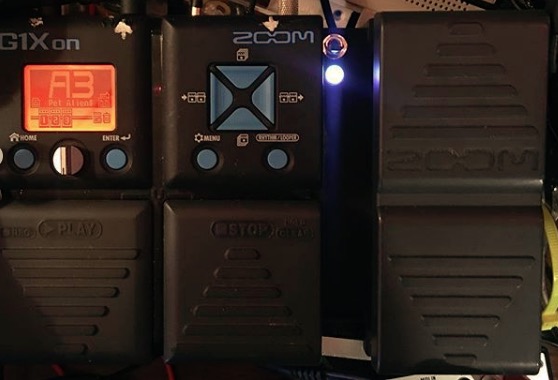
#sync with #drummachine so the pulse from the #po12 gets counted 8/16/32 times by a #digispark #arduino which then triggers a relay that stomps the #looper right in time on the #zoom #gx1on
some notes:
digispark arduino microcontroller programmed via usb. reed switch style relay in dip package. pulldown resistor on analog pin. to listen for the trigger pulse, we look for about a volt, so analogRead like 200. don’t forget a delay(30) so to you only count each trigger once.
i ended up using a stereo jack so that the audio from the po12 can pass in without and adaptor. although the audio is on the ring, it’s attached internally to the tip of the aux in, so it’s for sure audible on the tip of the out on the GX1on when connected to mono stuff. switch up is armed. when down, the color changes a little bit with each pulse it hears. if you arm it right after disarming it, (off/on quick enough) during this short fade of the armed color, that color changes to tell you it’s going to count 1/2/4 measures
code for arduino digispark digistump
// woz supposedly Jan 2019 - sync pulse counter for starting the looper in sync with drum machines and sequencers
#include <Arduino.h>
#include <Adafruit_NeoPixel.h>
Adafruit_NeoPixel strip = Adafruit_NeoPixel(1, 1, NEO_RGB + NEO_KHZ800);
#define relay 0
#define sw 2
#define in A0
#define thresh 100
#define btn 3
int c;
int arm;
int count;
int mes = 2;
int minc;
bool moved;
bool sent;
bool active;
int colorMap[3] = {25, 25, 25};
void setup() {
strip.begin();
strip.show();
strip.setBrightness(64);
strip.setPixelColor(0, strip.Color(colorMap[0], colorMap[1], colorMap[2]));
strip.show();
pinMode(relay, OUTPUT); //relay
pinMode(1, OUTPUT); //LED pin
pinMode(sw, INPUT_PULLUP); //switch
pinMode(btn, INPUT_PULLUP); //button
pinMode(in, INPUT); //sync input
digitalWrite(relay, LOW);
}
void loop() {
if (!digitalRead(sw)) {
inaction();
} else {
chillled();
}
}
void inaction() {
count = 0; // reset
sent = false;
moved = false;
minc = 0;
while (!digitalRead(sw)) {
if (!digitalRead(btn)) {
button();
}
if (analogRead(in) > thresh) {
count++;
moved = true;
if (count > 9) {
count = 9;
}
}
if (count == 0) {
arm++;
if (arm > 128) {
arm = 0;
}
if (arm > 64) {
if (mes == 0) {
strip.setPixelColor(0, strip.Color(128 - arm, 0, 0));
}
if (mes == 1) {
strip.setPixelColor(0, strip.Color(0, 128 - arm, 0));
}
if (mes == 2) {
strip.setPixelColor(0, strip.Color(0, 0, 128 - arm));
}
if (mes == 3) {
strip.setPixelColor(0, strip.Color(128 - arm, 128 - arm, 0));
}
} else {
if (mes == 0) {
strip.setPixelColor(0, strip.Color(arm, 0, 0));
}
if (mes == 1) {
strip.setPixelColor(0, strip.Color(0, arm, 0));
}
if (mes == 2) {
strip.setPixelColor(0, strip.Color(0, 0, arm));
}
if (mes == 3) {
strip.setPixelColor(0, strip.Color(arm, arm, 0));
}
}
}
if (count == 1) {
if (!sent) {
digitalWrite(relay, HIGH);
delay(15);
digitalWrite(relay, LOW);
sent = true;
}
strip.setPixelColor(0, strip.Color(128, 128, 128));
}
if (count == 2) {
strip.setPixelColor(0, strip.Color(255, 0, 0));
}
if (count == 3) {
strip.setPixelColor(0, strip.Color(255, 64, 0));
}
if (count == 4) {
strip.setPixelColor(0, strip.Color(255, 182, 0));
}
if (count == 5) {
strip.setPixelColor(0, strip.Color(0, 255, 0));
}
if (count == 6) {
strip.setPixelColor(0, strip.Color(0, 0, 255));
}
if (count == 7) {
strip.setPixelColor(0, strip.Color(255, 0, 255));
}
if (count == 8) {
strip.setPixelColor(0, strip.Color(32, 0, 64));
sent = false;
}
if (count == 9) {
if (((mes == 1) && (minc < 1)) || ((mes == 2) && (minc < 3)) || ((mes == 3) && (minc < 7))) {
minc++;
count = 1;
sent = true;
} else {
if (!sent) {
digitalWrite(relay, HIGH);
delay(15);
digitalWrite(relay, LOW);
sent = true;
for (arm = 0; arm < 255; arm++) {
strip.setPixelColor(0, strip.Color(255 - arm, 255 - arm, 255 - arm));
strip.show();
delay(2);
}
}
done();
}
}
strip.show();
if (moved) {
delay(30);
moved = false;
}
delay(2);
} //end while switch on
}
void chillled() {
while (digitalRead(sw)) {
if (!digitalRead(btn)) {
button();
}
if (analogRead(in) > thresh) {
if (arm == 64) {
arm = 32;
} else {
arm = 64;
}
if (mes == 0) {
strip.setPixelColor(0, strip.Color(arm, 0, 0));
}
if (mes == 1) {
strip.setPixelColor(0, strip.Color(0, arm, 0));
}
if (mes == 2) {
strip.setPixelColor(0, strip.Color(0, 0, arm));
}
if (mes == 3) {
strip.setPixelColor(0, strip.Color(arm, arm, 0));
}
delay(5);
}
strip.show();
}
}
void done() {
while (!digitalRead(sw)) {
if (analogRead(in) > thresh) {
c = random(0, 3);
if (random(2) >= 1) {
colorMap[c] += 25;
if (colorMap[c] > 128) {
colorMap[c] = 0;
}
} else {
colorMap[c] -= 25;
if (colorMap[c] < 0) {
colorMap[c] = 128;
}
}
if (!digitalRead(btn)) {
button();
}
strip.setPixelColor(0, strip.Color(colorMap[0], colorMap[1], colorMap[2]));
strip.show();
}
}
}
void button() {
int x = 0;
mes++;
if (mes > 3) {
mes = 0;
}
for (x = 0; x < 100; x++) { // loop to check for mes change
if (mes == 0) {
strip.setPixelColor(0, strip.Color(200 - x, 0, 0));
}
if (mes == 1) {
strip.setPixelColor(0, strip.Color(0, 200 - x, 0));
}
if (mes == 2) {
strip.setPixelColor(0, strip.Color(0, 0, 200 - x));
}
if (mes == 3) {
strip.setPixelColor(0, strip.Color(200 - x, 200 - x, 0));
}
strip.show();
delay(2);
}
}